Table Of Content
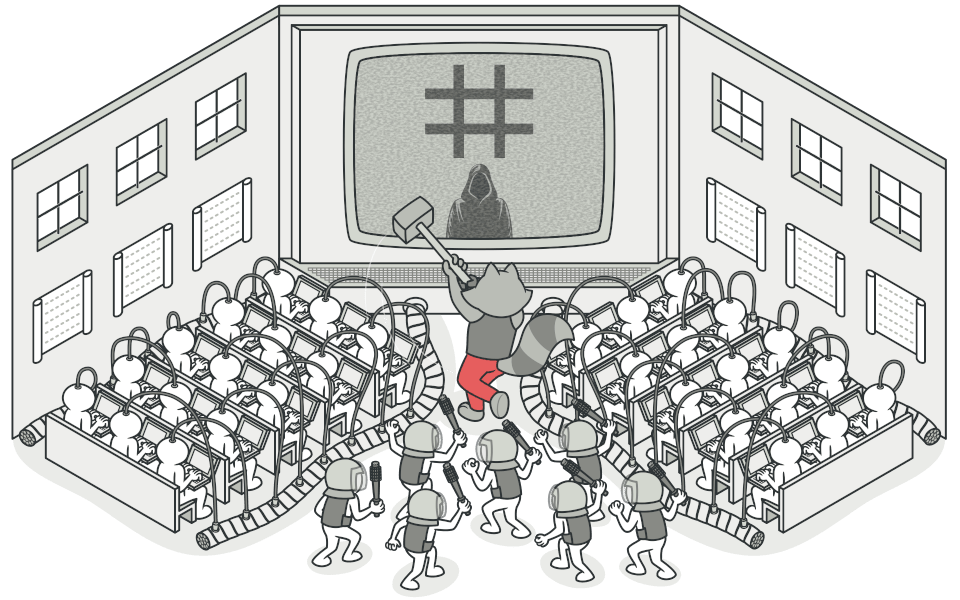
We will discuss how to implement the same using a .NET Application. We will compare the example with the UML diagram of the design pattern so that you will understand the concept very easily. Structural design patterns are a subset of design patterns in software development that focus on the composition of classes or objects to form larger, more complex structures. They help in organizing and managing relationships between objects to achieve greater flexibility, reusability, and maintainability in a software system.
Languages
In the singleton pattern, all the methods and instances are defined as static. The static keyword ensures that only one instance of the object is created, and you can call methods of the class without creating an object. The Abstract factory is a base class for concrete factory classes that generate or create a set of related objects. This base class contains the definition of a method for each type of object that will be instantiated.
The Essence Of Factory
Design patterns are used to solve these commonly occurring problems in the development phase so that we can minimize the problems after deployment. A design pattern suggests a specific implementation for the specific object-oriented programming problem. For example, if you want to ensure that only a single instance of a class exists, then you can use the Singleton design pattern which suggests the best way to create a class that can only have one object. Composite Method is structural design pattern, it’s used to compose objects into tree structures to represent part-whole hierarchies.
A real-world example of an Abstract factory design pattern using C#
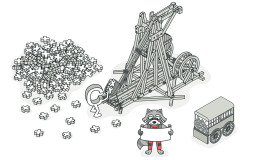
Practice as you learn with live code environments inside your browser. Lets you separate algorithms from the objects on which they operate. Connect and share knowledge within a single location that is structured and easy to search.
Design Patterns in C++ 20: Behavioral - Chain of Responsibility to Memento
The Creational Design Pattern deals with Object Creation and Initialization. The Creational Design Pattern gives the programmer more flexibility in deciding which objects need to be created for a given case. For example, if we have a huge project, a huge project means we have a lot of classes, and a lot of classes means we are dealing with many objects. So we need to create different objects (like new Customer(), new Product(), new Invoice(), etc.) based on some conditions.
Stellar Blade: Xion Walkthrough - Cans, Design Patterns, Body Cores - Push Square
Stellar Blade: Xion Walkthrough - Cans, Design Patterns, Body Cores.
Posted: Fri, 26 Apr 2024 03:30:37 GMT [source]
Button is the subject here, when the button is clicked, data gets changed. In short, Strategy Pattern helps us to dynamically change the way our components work as we write code and separates our concerns. If one day you want to remove fur from the tabby for your game, you can just change how displayBehavior is set in the Tabby class and the client won’t need to be notified of this change.
Proxy means ‘in place of’, representing’ or ‘in place of’ or ‘on behalf of’ are literal meanings of proxy and that directly explains Proxy Design Pattern. They are closely related in structure, but not purpose, to Adapters and Decorators. There are clear similarities between Staging and Production deploys, so you can create a template for both of them to use. You don’t want to migrate all your code to Client B, because you’ve heard that Client A will soon start supporting newer versions and Client B overall uses more memory than Client A. For example, it uses the name read instead of consume and uses the name write instead of produce. You can use this if you are sure you’ll use getInstance() at least one time in your application.
How to use the specification design pattern in C# - InfoWorld
How to use the specification design pattern in C#.
Posted: Thu, 09 Nov 2023 08:00:00 GMT [source]
Classification
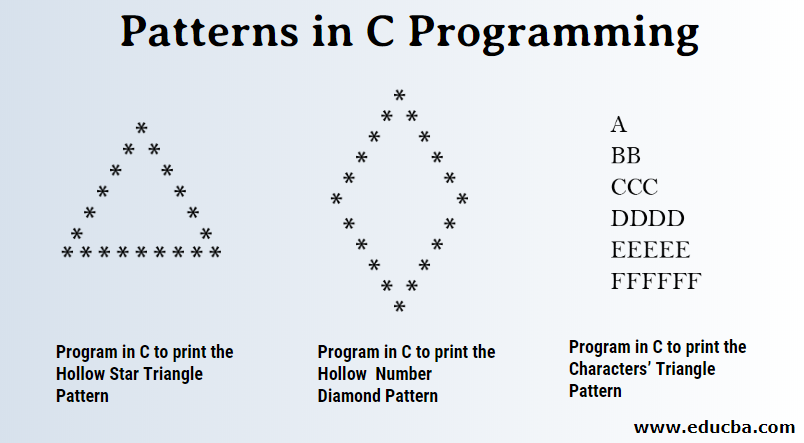
A design pattern in C++ is a generic repeatable solution to a frequently occurring problem in software design that is used in software engineering. It isn’t a complete design that can be written in code right away. It is a description or model for problem-solving that may be applied in a variety of contexts. Design patterns in C++ help developers create maintainable, flexible, and understandable code.
The Rhombus pattern is similar to the square pattern, just that we have to add spaces before each line and their count decreases progressively with rows. This pattern is the 180° flipped version of the Right Half Pyramid Pattern we discussed earlier. The Left Half Pyramid looks like a right-angled triangle with its hypotenuse facing the left. We can also print this pattern using a character, alphabets, or numbers.
Creational design patterns are a subset of design patterns in software development. They deal with the process of object creation, trying to make it more flexible and efficient. It makes the system independent and how its objects are created, composed, and represented.
The idea of a design pattern is an attempt to standardize what are already accepted best practices. In principle this might appear to be beneficial, but in practice it often results in the unnecessary duplication of code. It is almost always a more efficient solution to use a well-factored implementation rather than a "just barely good enough" design pattern. Design pattern in software engineering is a general, reusable solution to a commonly occurring problem in software design.
They help to abstract away object creation processes that spread across multiple classes. Design Patterns in Python introduces a powerful and essential concept in software development – design patterns. These patterns offer time-tested solutions to recurring design problems, providing a structured and efficient approach to building robust, scalable, and maintainable software systems in Python. The Strategy Design Pattern allows the behavior of an object to be selected at runtime. It is one of the Gang of Four (GoF) design patterns, which are widely used in object-oriented programming.